Laravel Livewire Starter Kits (All FREE)
Learn more about Laravel & Livewire and start secure and scalable projects on top of the starters presented in this article.
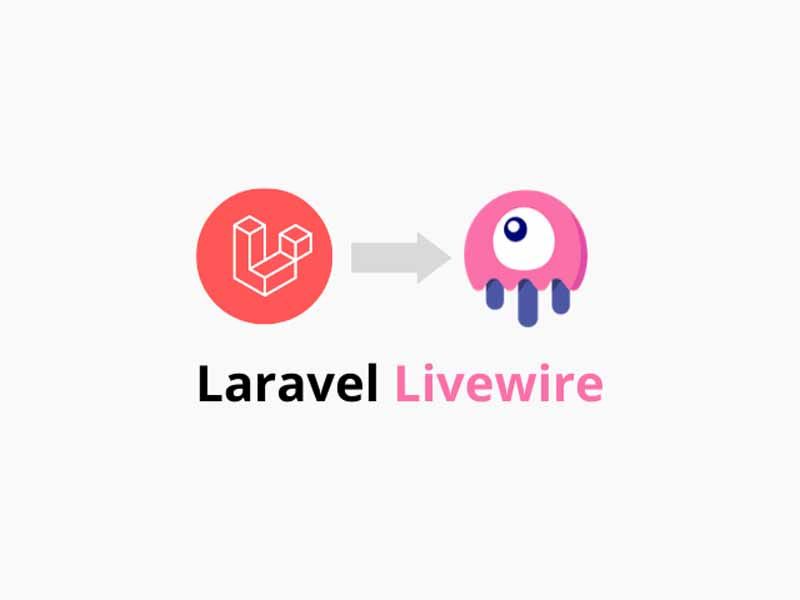
Hello! This article is a short introduction to Laravel and Livewire plus a short list of starter kits that anyone can use for free. For newcomers, Laravel and Livewire are two popular technologies in the world of web development, and they are often used together to build dynamic and interactive web applications.
✅ What is Laravel
Laravel is a free, open-source PHP web framework known for its elegance, simplicity, and developer-friendly syntax. It was created by Taylor Otwell and was first released in 2011.
Laravel follows the Model-View-Controller (MVC) architectural pattern and provides a wide range of features and tools to simplify web application development. Here are the key features of Larave:
- Eloquent ORM: Laravel includes an elegant and intuitive ActiveRecord implementation called Eloquent for working with databases.
- Blade Templating: A lightweight yet powerful templating engine for creating dynamic views.
- Artisan Console: A command-line tool for automating various development tasks.
- Authentication and Authorization: Built-in support for user authentication and role-based access control.
- Routing: Easy and flexible routing system for defining web and API routes.
- Middleware: Middleware layers for filtering HTTP requests entering your application.
- Database Migrations and Seeding: Tools for managing database schemas and populating them with sample data.
- Testing: Comprehensive testing support with PHPUnit.
Community: Laravel has a vibrant and active community, which means extensive documentation, packages, and community-driven resources are available.
✅ What is Livewire
Livewire is a full-stack framework for building dynamic web applications in Laravel using only PHP and Blade templates. It was created by Caleb Porzio and introduced a new way of building interactive interfaces without the need for JavaScript. Livewire can be used for:
- Real-Time Updates: Livewire enables real-time updates in web applications without writing custom JavaScript code.
- Server-Side Rendering: The majority of the code runs on the server, reducing the need for complex client-side logic.
- Components: Developers can create reusable components using Livewire, which encapsulate both the HTML and PHP logic.
- Validation and Form Handling: Livewire simplifies form handling and validation.
- Hooks and Actions: Allows developers to respond to user interactions and perform actions on the server.
Integration with Laravel: Livewire seamlessly integrates with Laravel applications, making it easy to add dynamic, interactive features to your Laravel-powered websites.
Besides the above, the Livewire Community has gained popularity in the Laravel community, leading to the creation of numerous packages, tutorials, and resources.
✅ Coding Samples
To move forward from theory, here are some basic coding samples to give you an idea of how Laravel and Livewire work together to create dynamic web applications. These examples assume you have a basic understanding of Laravel and have set up a Laravel project.
✅ Laravel Route and Livewire Component
Let's start with a simple example of setting up a route in Laravel and creating a Livewire component to display a counter.
👉 Create a Livewire Component
First, create a new Livewire component. In your terminal, run:
php artisan make:livewire Counter
This command will create a new Livewire component named Counter
in the app/Http/Livewire
directory.
👉 Edit the Counter Component
Open the app/Http/Livewire/Counter.php
file and define a property and a method to increment the counter:
// app/Http/Livewire/Counter.php
namespace App\Http\Livewire;
use Livewire\Component;
class Counter extends Component
{
public $count = 0;
public function increment()
{
$this->count++;
}
public function render()
{
return view('livewire.counter');
}
}
👉 Create a Blade View
Next, create a Blade view for the Livewire component. Create a new Blade file at resources/views/livewire/counter.blade.php
and add the following code:
<!-- resources/views/livewire/counter.blade.php -->
<div>
<button wire:click="increment">Increment</button>
<p>Count: {{ $count }}</p>
</div>
👉 Create a Route
In your routes/web.php
file, define a route that renders the Livewire component:
// routes/web.php
use App\Http\Livewire\Counter;
Route::get('/counter', Counter::class);
👉 View in Browser
Run the Laravel development server:
php artisan serve
Visit http://localhost:8000/counter
in your web browser, and you should see the counter component with an "Increment" button.
✅ Livewire Form Validation
Here's an example of how Livewire can handle form validation in a Laravel application:
👉 Create a Livewire Component
Create a new Livewire component for a contact form:
php artisan make:livewire ContactForm
👉 Edit the ContactForm Component
In app/Http/Livewire/ContactForm.php
, define properties for form fields, add validation rules, and handle form submission:
// app/Http/Livewire/ContactForm.php
namespace App\Http\Livewire;
use Livewire\Component;
use Illuminate\Support\Facades\Mail;
use App\Mail\ContactMail;
class ContactForm extends Component
{
public $name;
public $email;
public $message;
protected $rules = [
'name' => 'required',
'email' => 'required|email',
'message' => 'required|min:10',
];
public function submit()
{
$this->validate();
// Send email
Mail::to('contact@example.com')->send(new ContactMail($this->name, $this->email, $this->message));
// Reset form fields
$this->reset();
}
public function render()
{
return view('livewire.contact-form');
}
}
👉 Create a Blade View
Create a Blade view for the contact form at resources/views/livewire/contact-form.blade.php
:
<!-- resources/views/livewire/contact-form.blade.php -->
<form wire:submit.prevent="submit">
<div>
<label for="name">Name</label>
<input type="text" wire:model="name">
@error('name') <span>{{ $message }}</span> @enderror
</div>
<div>
<label for="email">Email</label>
<input type="email" wire:model="email">
@error('email') <span>{{ $message }}</span> @enderror
</div>
<div>
<label for="message">Message</label>
<textarea wire:model="message"></textarea>
@error('message') <span>{{ $message }}</span> @enderror
</div>
<button type="submit">Submit</button>
</form>
👉 Create a Route
In routes/web.php
, define a route that renders the Livewire contact form component:
// routes/web.php
use App\Http\Livewire\ContactForm;
Route::get('/contact', ContactForm::class);
👉 View in Browser
Run the Laravel development server and visit http://localhost:8000/contact
in your web browser. You should see the contact form with validation and submission handled by Livewire.
These examples demonstrate how to create basic functionality using Laravel and Livewire. You can build more complex applications by expanding on these concepts and utilizing the rich features of Laravel and the interactivity provided by Livewire.
✅ Laravel Livewire Starters
This section presents a curated list of open-source starters powered by Laravel & Livewire, that anyone can use for hobby or commercial projects.
👉 Laravel Livewire Soft Dashboard
Soft UI Dashboard Laravel Livewire has dozens of handcrafted UI elements tailored for Bootstrap 5 and an out-of-the-box Laravel backend.
The Livewire integration allows you to build dynamic interfaces more easily without leaving the comfort of your favorite framework.
- 👉 Laravel Livewire Soft Dashboard - product page
- 👉 More Laravel & Livewire starters provided by Creative-Tim
If you combine this even further with Alpine.js, you get the perfect combo for kickstarting your next project.
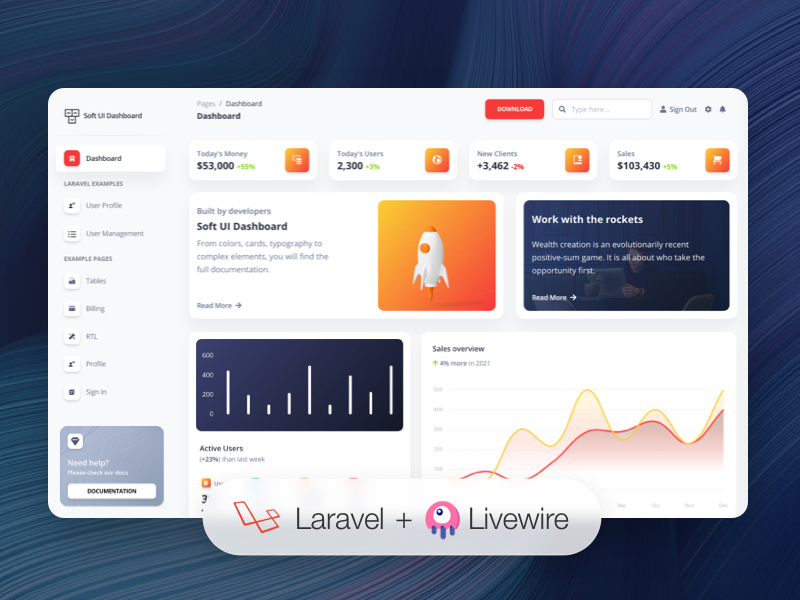
👉 Laravel Livewire Material Dashboard
Material Dashboard Laravel Livewire combines a fresh, new design inspired by Google's Material Design 2 with a Laravel backend and Livewire.
In short, it's the ultimate full-stack resource to help developers build complex apps faster.
- 👉 Laravel Livewire Material Dashboard - product page
- 👉 More Laravel & Livewire starters provided by Creative-Tim
Material Dashboard 2 Laravel Livewire comes with Laravel authentication, registration, and user profile, a must-have for any app you're going to build. Add in Livewire and you have everything you need to get started right away.
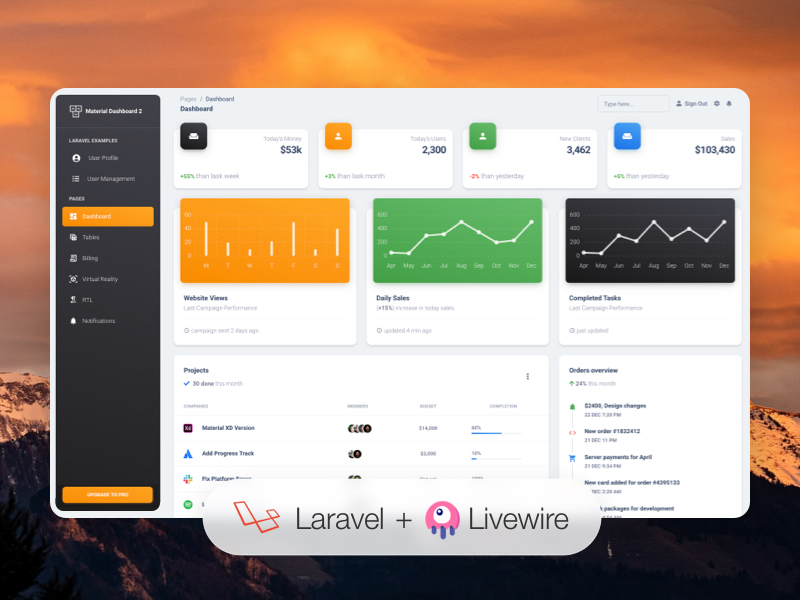
👉 Soft UI Dashboard TALL
With Soft UI Dashboard TALL Stack, you're getting a fast and easy way to build Laravel apps, easily style them with Tailwind, and write Livewire components with a dash of Alpine.js. Even more, Soft UI Dashboard TALL Stack comes with:
- 70 handcrafted UI components. From buttons and inputs to navbars and cards, everything is designed to create the best user experience
- 7 example pages to give you a taste of what you can build with Soft UI Dashboard TALL Stack and 1 customized plugin
- Out-of-the-box authentication system, register, and user profile editing features built with Laravel
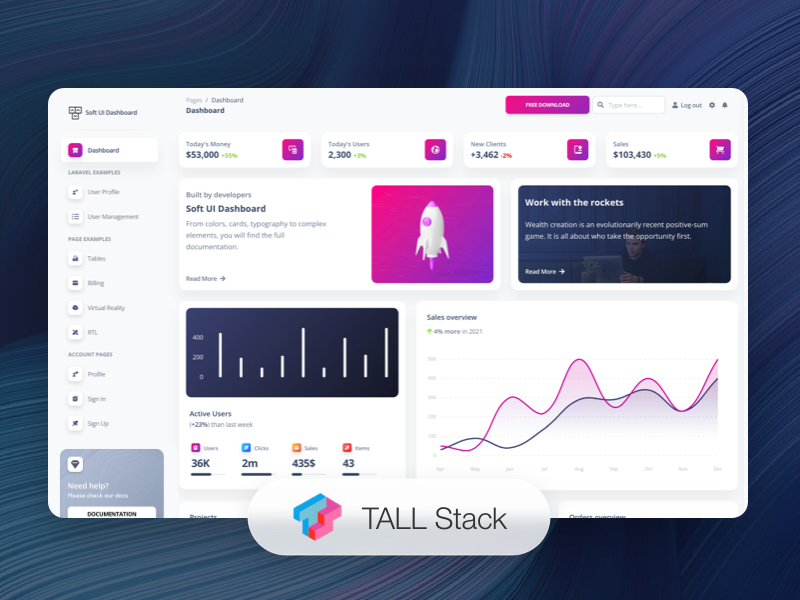
✅ In Summary
Laravel is a powerful PHP web framework that provides the foundation for building web applications, while Livewire is a library that enhances Laravel by enabling the creation of interactive, real-time web interfaces using PHP and Blade templates.
These examples demonstrate how to create basic functionality using Laravel and Livewire. You can build more complex applications by expanding on these concepts and utilizing the rich features of Laravel and the interactivity provided by Livewire.
✅ Resources
- 👉 Access AppSeed and start your next project
- 👉 Deploy Projects on Aws, Azure, and DO via DeployPRO
- 👉 Create landing pages with Simpllo, an open-source site builder
- 👉 Django App Generator - A 2nd generation App Builder