Node JS for Beginners - Practical Guide
A comprehensive introduction to Node JS for Beginners - With Free Samples.
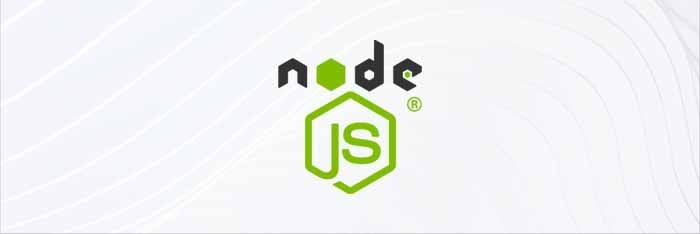
Hello! In this article, we will set up a simple NodeJS application that will serve a nice web page styled with Bootstrap 5
. In the end, we will use Docker
to make the project deployable in production without any configuration hassle. For newcomers, NodeJS is a popular technology that uses JavaScript
to implement performant backend features like authentication, database queries, and static assets management.
Thanks for reading!
Topics covered:
- 👉  What is
JavaScript
- 👉 Â
Setting up
the environment - 👉  Choose a
code editor
- 👉  Code a simple
NodeJS
Project - 👉  Integrate
Docker
(for deployment) - 👉  Popular
NodeJS Modules
- 👉 Â
Free NodeJS Starters
: Berry, Datta Able, Purity (Chakra UI) - 👉
Free Resources
& Links
✨ What is JavaScript
JavaScript
has become a really hot topic in the programming language. At the moment this article is written, JavaScript is known to be the most popular language in the world with over 69.7% of use in software development.
JavaScript
is well known for the development of web apps but JavaScript can be used for a variety of purposes also: for native Mobile Applications we can use frameworks like React Native or Ionic, for Back-end Web Development we can use NodeJS, or Machine Learning.
For more information regarding this popular programming language, feel free to access these free resources:
✨ Setting up
the environment
To get started, please head to the Node.js official website https://nodejs.org/en/ and download the stable version of Node.js that is recommended for most users. As of now, the recommended version is 16.14.2 as seen in the figure below (highlighted with a red border):
Once downloaded, click on the installation file to install it on your PC. To ensure that Node.js is installed on your PC, open a terminal window and type:
$ node -v
If it is installed correctly, the above command would return the version of the Node.js that you just installed which in my case would be 16.14.2
.
✨ Choose a Code Editor
There are many code editors/IDEs like IntelliJ IDEA
and Sublime Text
that you can use for developing applications in NodeJS
and it all comes down to personal preference to a great extent. However, Visual Studio Code
(VS Code) is the most famous among developers according to the official StackOverflow survey. For the purpose of this tutorial, I would also be using VS code not only because it is well known but because it is my personal favorite as well.
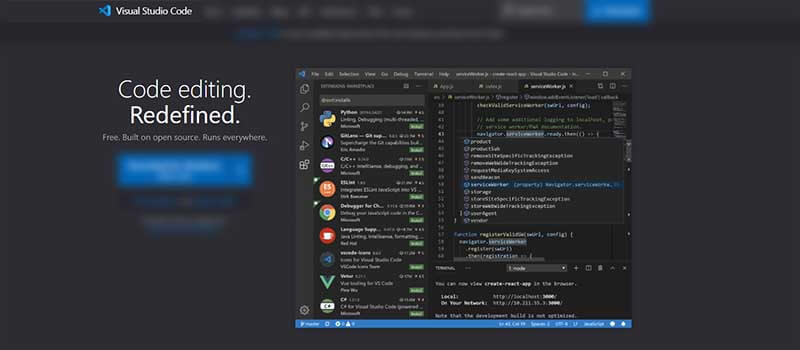
✨ Code a simple NodeJS
Project
First thing is first, we name our project. Go to file explorer on your PC and create a folder with the name of the project that you want. You can create this folder anywhere. For this example, I have created a folder and named it blog-sample-nodejs-introduction
as seen in the figure below:
Now open VS code (kindly install it if you already haven’t by heading to https://code.visualstudio.com/ and downloading the stable build). In the VS code, open the folder that you just created, and now this will act as your workspace. All the files and code will be written inside this workspace.
Now, before we start creating files and begin coding, we need to perform one more step. And, that step is to run the following command:
$ npm init
This command will create a file called package.json
and it is a file that is used to set up new or existing npm packages.
You can think of an npm package
as a module that provides several functions and methods to ease our development process. When you will run the command npm init
, it will ask for your input on various metadata like the name of the package, etc. which you can give according to your personal preference except for the attribute main
as shown in the figure below.
For the “main” attribute, you need to enter the name of the entry file from where our backend server will begin running. We haven’t created that file yet, but I have named it server.js as the name should clearly reflect the purpose of the file and the code inside it. Here is how my package.json
looks:
{
"name": "nodejs-article",
"version": "1.0.0",
"description": "",
"main": "server.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1",
"start": "node server.js"
},
"author": "",
"license": "ISC",
"dependencies": {
"express": "^4.17.3"
}
}
Installing the package
Before we can begin writing our first lines of code for our NodeJS
backend server we need to install a module called Express
which is a minimal and flexible NodeJS
web application framework that provides a robust set of features for web and mobile applications.
Writing the code
Create a new file called server.js
in the same directory in which you just created package.json
. In the server.js
, paste the following code:
const express = require('express');
const app = express();
const port = 3000;
app.get("/api", (request,response)=>{
response.send("Hello World")
})
app.listen(port, (req,res)=>{
console.log(`Listening at port ${port}` )
})
The above code simply responds with Hello World
when we try to access the URL: localhost:3000/api
. Once you have pasted the code run the following command in VS code terminal to run the server:
$ node server.js
In the terminal you should be able to see the following output:
$ node server.js
Listening at port 3000
You should see the following result on your web browser:
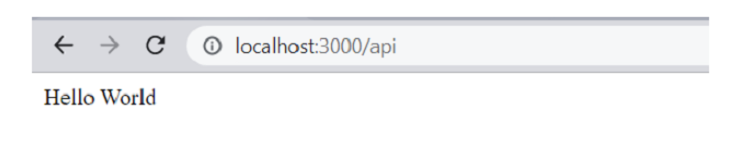
You have just managed to set up your first NodeJS
Backend server, but hey what if you want to show some beautiful web page to your users using your NodeJS
? This is exactly what we will be doing next.
The express.static
is a function inside express that enables the server to serve static files like HTML
, images
, CSS
, JavaScript
, etc. In our case, we are serving the index.html
and the assets folder together (the most simple case possible).
The final code of your server.js file should look like this:
const express = require('express');
const app = express();
const port = 3000;
app.use(express.static('public')) // <-- NEW
app.get("/api", (request,response)=>{
response.send("Hello World")
})
app.listen(port, (req,res)=>{
console.log(`Listening at port ${port}` )
})
Restart the server and open localhost:3000
in the browser. You shall see a sophisticated and eye-catching web page as shown in the figure below:
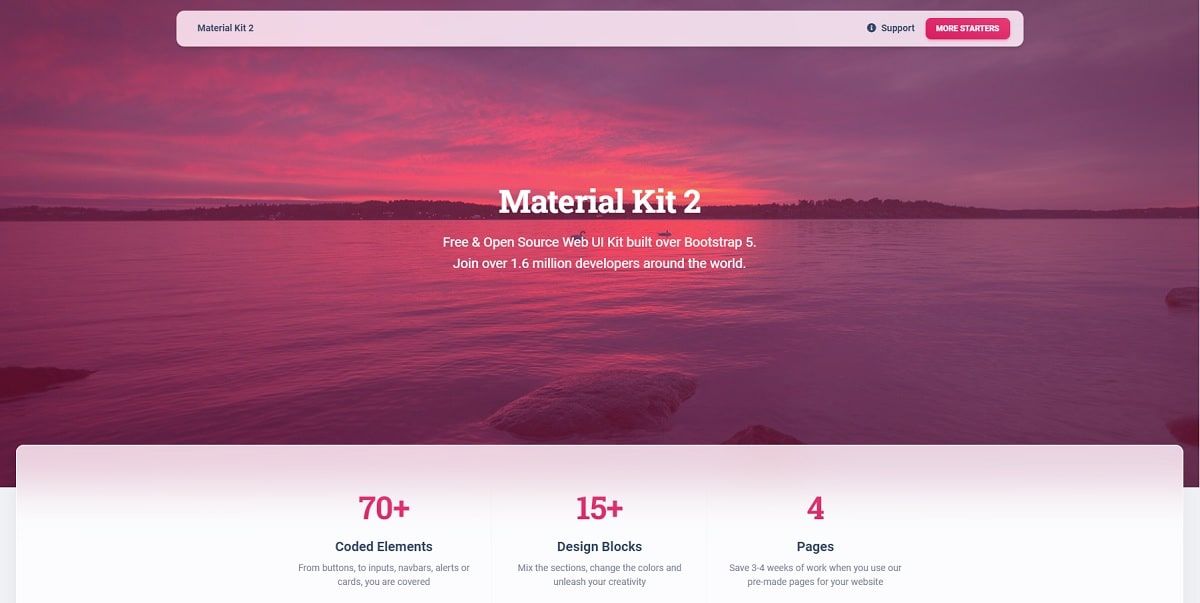
Once this design is integrated, we can move forward and add Docker support.
✨ Integrate Docker
Docker is a service that uses OS-level virtualization to package software into an isolated environment called containers. In other words, Docker is a containerization platform, that combines application source code with OS libraries and dependencies and packages it into a single unit that can be run in any environment.
Running the project with Docker
The prerequisite for running the application with Docker is that first, you need to install Docker Desktop on your PC.
Head to https://www.docker.com/products/docker-desktop/, and install Docker according to your PC environment. Â Once installed, verify the installation by typing the following command into your terminal:
$ docker -v
If the above command runs without errors, that means the Docker was installed successfully and we can use it to start the NodeJS
sample via a single command:
$ docker-compose up --build
The main advantage of using Docker is the isolated environment execution for the project and also the fact that we've executed a single command. Of course, under a Docker set up we can execute more commands and complex deployment scripts but this is not the case for our super simple project.
✨ Popular Node JS Extensions
Our NodeJS project is fairly simple but once the projects are getting bigger and more features are required to be implemented, we might need more modules and libraries that make our life easier during the coding phase. Well, this section mentions a few popular NodeJS libraries widely used in (almost) every NodeJS project.
multer
Multer is a NodeJS
Middleware for handling multipart/form-data, which is primarily used for uploading files.
mysql
The official NodeJS
driver for MySql. It is written in JavaScript, does not require compiling, and is 100% MIT licensed.
dotenv
dotenv
is a zero-dependency module that loads environment variables from a .env
file into process.env.
mongoose
Mongoose is a MongoDB object modeling tool designed to work in an asynchronous environment. Mongoose supports both promises and callbacks.
nodemon
nodemon
is a tool that helps develop NodeJS
based applications by automatically restarting the node application when file changes in the directory are detected.
Those that patiently follow up on the content, can play with a few open-source Starters and Templates crafted on top of NodeJS
and Express
.
✨ React Node JS Berry
Berry is a creative React Dashboard built using the Material-UI. It is meant to be the best User Experience with highly customizable feature-riched pages. It is a complete game-changer React Dashboard with an easy and intuitive responsive design on retina screens or laptops.
- 👉 React Node JS Berry - product page
- 👉 React Node JS Berry - LIVE Demo
The product comes with a simple JWT authentication flow: login
/register
/logout
powered by an open-source Node JS API Backend via Passport
Library.
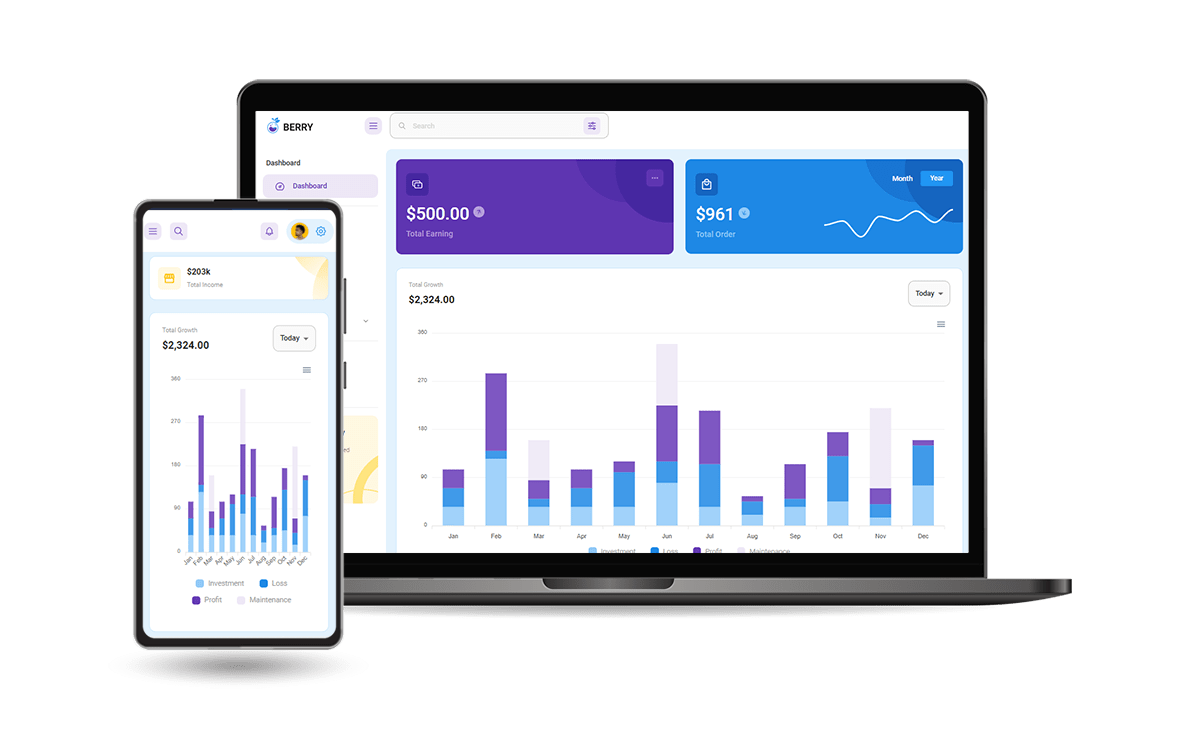
✨ React Node JS Datta Able
Datta Able is a colorful free React Admin Dashboard crafted by CodedThemes. It comes with high feature-rich pages and components with fully developer-centric code.
- 👉 React Node JS Datta Able - product page
- 👉 React Node JS Datta Able - LIVE Demo
The product comes with a simple JWT authentication flow: login
/register
/logout
powered by an open-source Node JS API Backend via Passport
Library.
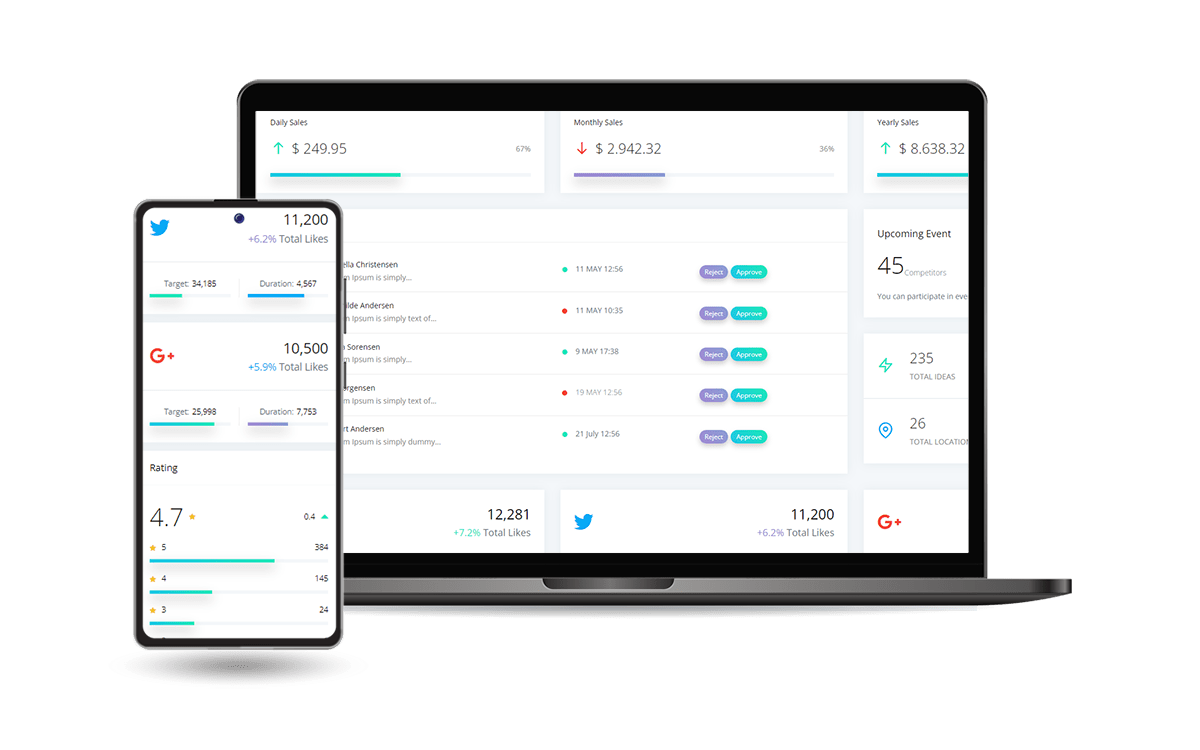
✨ React NodeJS Purity
A colorful free React Admin Dashboard crafted by Creative-Tim on top of Chakra UI. Designed for those who like modern UI elements and beautiful websites.
- 👉 React Purity Dashboard - product page
- 👉 React Purity Dashboard - LIVE Demo
Made of hundred of elements, designed blocks, and fully coded pages, Purity UI Dashboard is ready to help you create stunning websites and web apps.
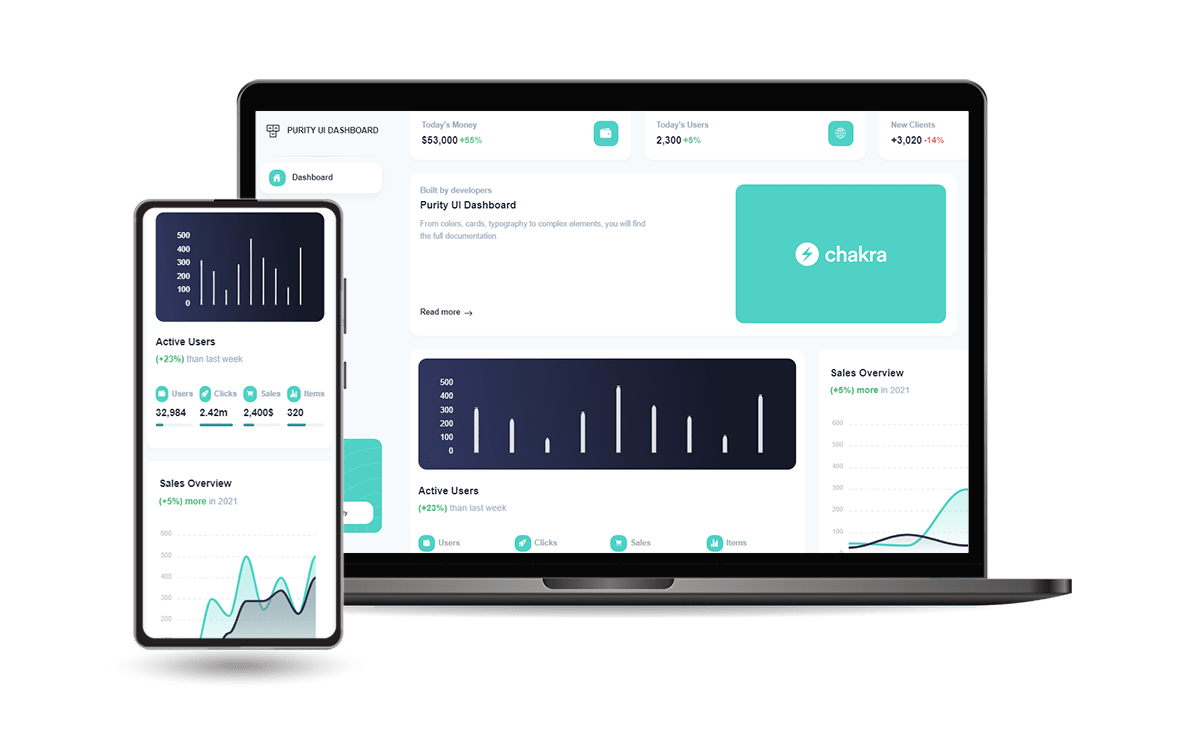