Spike NextJS
Free dashboard starter powered by NextJS and MUI - Spike Template is provided by WrapPixel Agency.
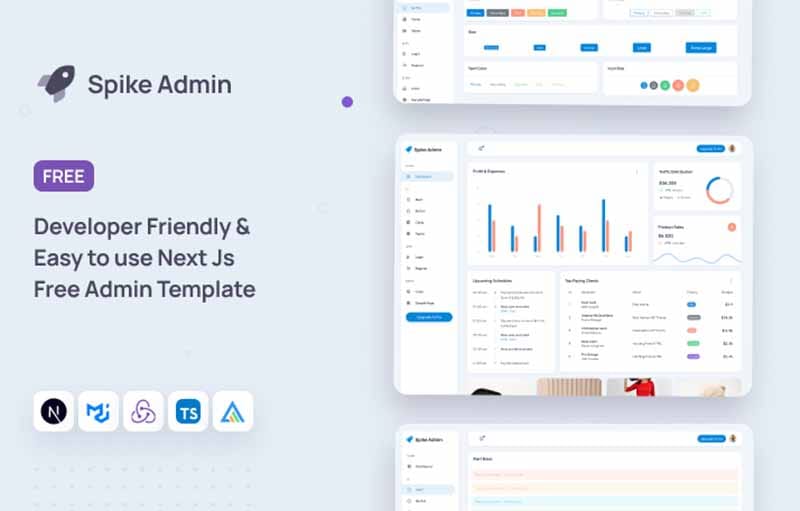
Hello! This article presents Spike, a modern NextJs Dashboard Template crafted and released for free by the WrapPixel agency. This starter provides a simple, intuitive codebase that can be easily extended to a fully-fledged dashboard application.
- 👉 Spike NextJS - product page (contains download link)
- 👉 More Free Templates provided by WrapPixel
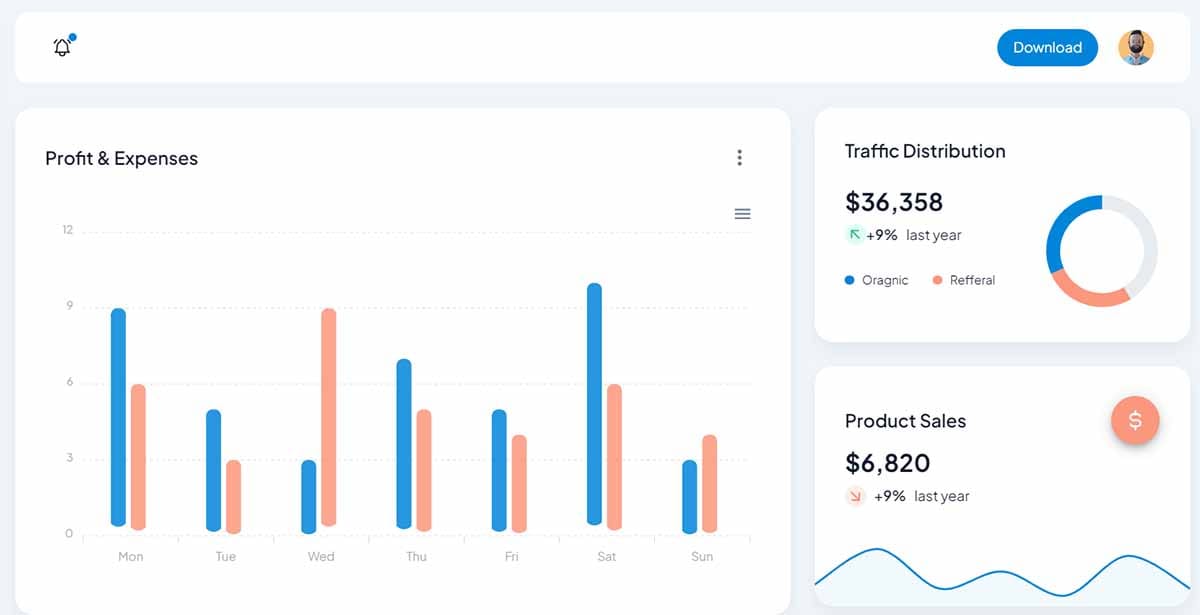
This free product is built on top of a modern design powered by NextJS and MUI, the most popular components library for React.
It allows you to create stunning backend applications and more. It comes with ready-to-use UI Blocks and elements to help level up the design and aesthetics of your project.
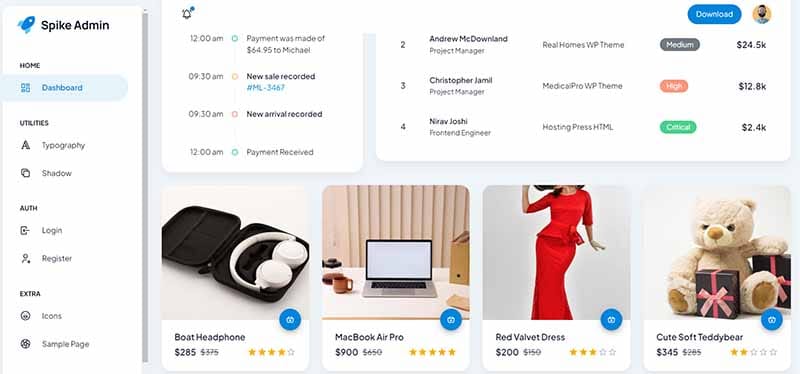
This free version comes with an elegant grid design that helps you play around with the look and feel of the web app the way you want.
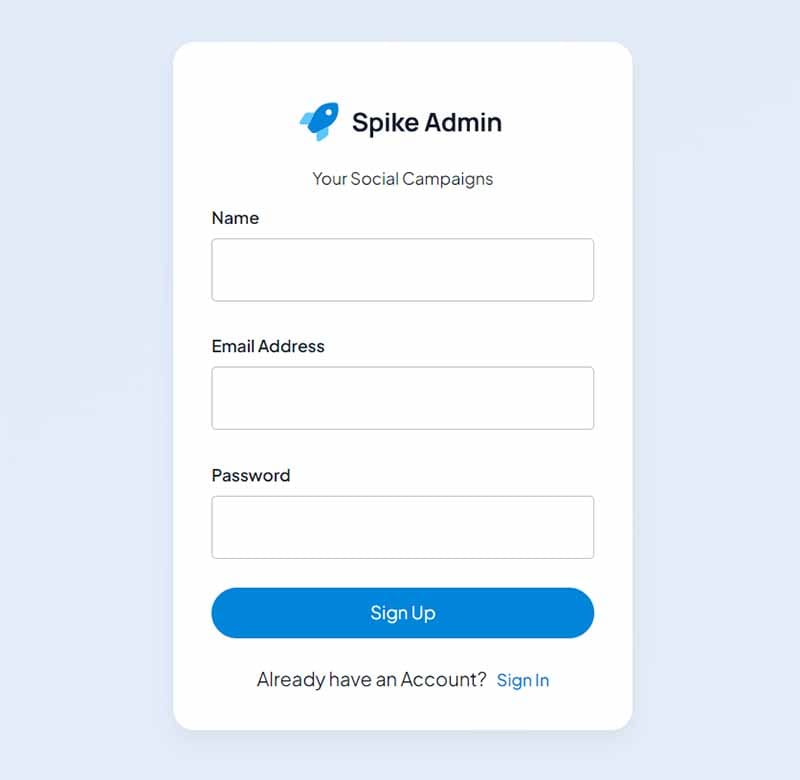
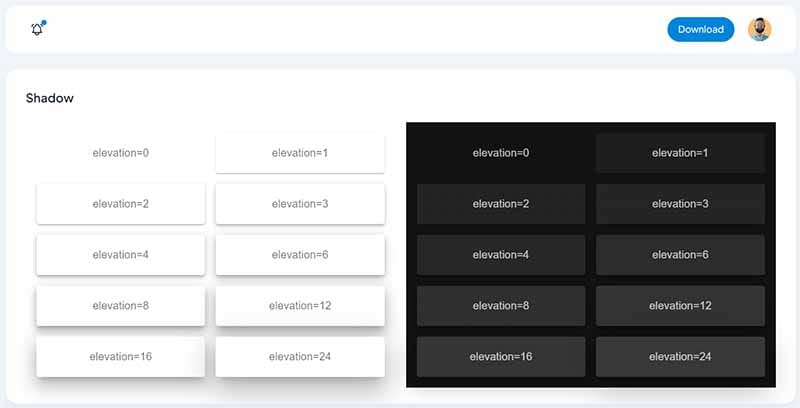
For those new to NextJS and MUI, here is a short introduction to this powerful stack.
Integrate MUI in NextJS
Next.js is a popular React framework used for building web applications. It simplifies the process of setting up React applications by providing features like server-side rendering, automatic code splitting, routing, and more.
Material-UI (MUI) is a React UI framework that implements Google's Material Design principles. It offers a collection of ready-to-use components for building sleek and modern user interfaces in React applications.
Integrating Material-UI components in a Next.js project involves several steps:
Setting Up a Next.js Project
If you haven't created a Next.js project yet, you can use create-next-app
to generate a new one:
npx create-next-app my-nextjs-app
Installing Material-UI
Install Material-UI and its dependencies in your Next.js project:
npm install @mui/material @emotion/react @emotion/styled
Setting Up a Custom Document
Create a _document.js
file in the pages
directory. This file allows you to customize the initial HTML document used for server-side rendering:
// pages/_document.js
import React from 'react';
import Document, { Html, Head, Main, NextScript } from 'next/document';
import { ServerStyleSheets } from '@mui/styles';
export default class MyDocument extends Document {
render() {
return (
<Html lang="en">
<Head>
{/* Other head elements */}
</Head>
<body>
<Main />
<NextScript />
</body>
</Html>
);
}
}
Setting Up Custom App
Create a _app.js
file in the pages
directory. This file allows you to initialize pages:
// pages/_app.js
import React from 'react';
import { ThemeProvider } from '@mui/material/styles';
import CssBaseline from '@mui/material/CssBaseline';
import theme from '../src/theme';
export default function MyApp({ Component, pageProps }) {
React.useEffect(() => {
// Remove the server-side injected CSS
const jssStyles = document.querySelector('#jss-server-side');
if (jssStyles) {
jssStyles.parentElement.removeChild(jssStyles);
}
}, []);
return (
<ThemeProvider theme={theme}>
<CssBaseline />
<Component {...pageProps} />
</ThemeProvider>
);
}
Using Material-UI Components
Now you can use Material-UI components throughout your Next.js application:
import React from 'react';
import Button from '@mui/material/Button';
const MyPage = () => {
return (
<div>
<h1>Welcome to My Next.js App</h1>
<Button variant="contained" color="primary">
Click me
</Button>
</div>
);
};
export default MyPage;
This is a basic setup to integrate Material-UI components into a Next.js application. You can explore more complex components, theming options, and customization provided by Material-UI based on your project requirements.
✅ Resources
Thanks for reading! For more resources and support, feel free to access: ​
- 👉 Access AppSeed and start your next project
- 👉 Deploy Projects on Aws, Azure, and DO via DeployPRO
- 👉 Create landing pages with Simpllo, an open-source site builder
- 👉 Django App Generator - A 2nd generation App Builder